Request Reply
Camel supports the Request Reply from the EIP patterns.
When an application sends a message, how can it get a response from the receiver?
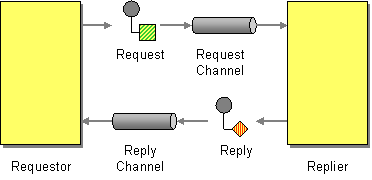
Send a pair of Request-Reply messages, each on its own channel.
Camel supports Request Reply by the Exchange Pattern on a Message which can be set to InOut
to indicate a request/reply message. Camel Components then implement this pattern using the underlying transport or protocols.
For example, when using JMS with InOut
the component will by default perform these actions:
-
create by default a temporary inbound queue
-
set the
JMSReplyTo
destination on the request message -
set the
JMSCorrelationID
on the request message -
send the request message
-
consume the response and associate the inbound message to the belonging request using the
JMSCorrelationID
(as you may be performing many concurrent request/responses). -
continue routing when the reply is received and populated on the Exchange
See the related Event Message. |
Using endpoint URI
If you are using a component which defaults to InOnly
you can override the Exchange Pattern for a consumer endpoint using the pattern property.
foo:bar?exchangePattern=InOut
This is only possible on endpoints used by consumers (i.e., in <from> ). |
In the example below the message will be forced as a request reply message as the consumer is in InOut
mode.
-
Java
-
XML
from("jms:someQueue?exchangePattern=InOut")
.to("bean:processMessage");
<route>
<from uri="jms:someQueue?exchangePattern=InOut"/>
<to uri="bean:processMessage"/>
</route>
Using setExchangePattern EIP
You can specify the Exchange Pattern using setExchangePattern
in the DSL.
-
Java
-
XML
from("direct:foo")
.setExchangePattern(ExchangePattern.InOut)
.to("jms:queue:cheese");
<route>
<from uri="direct:foo"/>
<setExchangePattern pattern="InOut"/>
<to uri="jms:queue:cheese"/>
</route>
When using setExchangePattern
then the Exchange Pattern on the Exchange is changed from this point onwards in the route.
This means you can change the pattern back again at a later point:
from("direct:foo")
.setExchangePattern(ExchangePattern.InOnly)
.to("jms:queue:one-way");
.setExchangePattern(ExchangePattern.InOut)
.to("jms:queue:in-and-out")
.log("InOut MEP received ${body}")
Using setExchangePattern to change the Exchange Pattern is often only used in special use-cases where you must force to be using either InOnly or InOut mode when using components that support both modes (such as messaging components like ActiveMQ, JMS, RabbitMQ etc.) |
JMS component and InOnly vs. InOut
When consuming messages from JMS a Request Reply is indicated by the presence of the JMSReplyTo
header. This means the JMS component automatic detects whether to use InOnly
or InOut
in the consumer.
Likewise, the JMS producer will check the current Exchange Pattern on the Exchange to know whether to use InOnly
or InOut
mode (i.e., one-way vs. request/reply messaging)